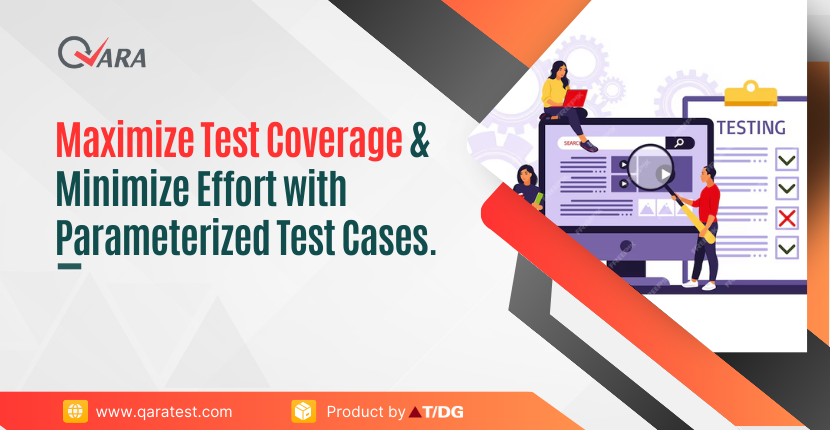
Parameterization of Test Cases: Enhancing Flexibility and Efficiency in Software Testing
What is Parameterization in Testing?
Why Parameterization is necessary?
- Increased Test Coverage: Parameterization enables the execution of a single test case with multiple data sets, ensuring that various input combinations are tested. This leads to more comprehensive test coverage and helps identify defects that may not be apparent with a limited set of inputs.
- Reduced Redundancy: By using parameterized test cases, testers can avoid duplicating test scripts for different input values. This reduces redundancy and simplifies test maintenance, as changes to the test logic need to be made only once.
- Improved Efficiency: Parameterization streamlines the software testing process by allowing the reuse of test cases with different data sets. This reduces the time and effort required to create and manage test cases, leading to more efficient testing cycles.
- Enhanced Flexibility: Parameterized test cases can easily accommodate new input data without requiring modifications to the test scripts. This flexibility is particularly useful in agile and continuous integration environments, where requirements and data sets may change frequently.
- Better Test Data Management: Parameterization promotes the use of external data sources, such as spreadsheets or databases, to manage test data. This centralized approach to test data management ensures consistency and simplifies data updates.
How to do Parameterization?
- Identify Parameters: The first step is to identify the input values that can be parameterized. These are typically the variables that change across different test scenarios. For example, in a login test case, the parameters could be the username and password.
- Define Data Sets: Once the parameters are identified, the next step is to define the data sets that will be used for software testing. This can be done using various data sources, such as spreadsheets, CSV files, databases, or data tables within the test management tool. Each data set should include values for all the identified parameters.
- Configure Test Scripts: The test scripts need to be configured to accept parameters and use them during execution. This involves replacing hardcoded values with variables and ensuring that the test scripts can dynamically retrieve and use the parameter values from the data sets.
- Execute Parameterized Tests: With the test scripts configured, the parameterized tests can be executed. The test management tool or automation framework will iterate through the data sets, running the test case with each set of input values.
- Username
- Password
Username | Password |
user1@example.com | pass123 |
user2@example.com | pass456 |
user3@example.com | pass789 |
import csv
from selenium import webdriver
# Function to read data from CSV file
def read_test_data(file_path):
data = []
with open(file_path, 'r') as file:
reader = csv.DictReader(file)
for row in reader:
data.append(row)
return data
# Test script using parameterized data
def test_login(username, password):
driver = webdriver.Chrome()
driver.get("https://example.com/login")
# Enter username and password
driver.find_element_by_id("username").send_keys(username)
driver.find_element_by_id("password").send_keys(password)
# Click login button
driver.find_element_by_id("loginButton").click()
# Add assertions to verify login success or failure
# ...
driver.quit()
# Main function to execute parameterized tests
if __name__ == "__main__":
test_data = read_test_data("login_data.csv")
for data in test_data:
test_login(data['Username'], data['Password'])
Best Practices for Parameterization in software testing
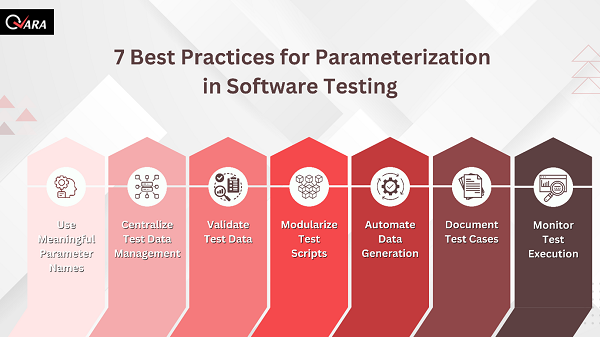
- Use Meaningful Parameter Names: Choose descriptive names for parameters to make the test scripts more readable and maintainable. Avoid using generic names like “param1” or “value1”.
- Centralize Test Data Management: Store test data in a centralized location, such as a database or a shared spreadsheet, to ensure consistency and simplify updates. This also facilitates collaboration among team members.
- Validate Test Data: Ensure that the test data is valid and covers all possible scenarios, including edge cases and negative cases. This helps identify defects that may not be apparent with typical input values.
- Modularize Test Scripts: Break down test scripts into smaller, reusable modules that can be parameterized independently. This promotes code reuse and simplifies maintenance.
- Automate Data Generation: Use data generation tools or scripts to create large sets of test data automatically. This is particularly useful for performance testing and stress testing, where a large volume of data is required.
- Document Test Cases: Clearly document the parameterized test cases, including the parameters, data sets, and expected outcomes. This helps ensure that the test cases are understandable and maintainable.
- Monitor Test Execution: Regularly monitor the execution of parameterized tests to identify any issues or anomalies. This helps ensure that the tests are running as expected and that the test data is being used correctly.
Challenges and Solutions in Parameterization
Parameterization of test cases is a powerful technique that enhances the flexibility, efficiency, and coverage of software testing. By allowing test cases to be executed with different sets of input data, parameterization reduces redundancy, improves test coverage, and simplifies test maintenance. Implementing parameterization involves identifying parameters, defining data sets, configuring test scripts, and executing parameterized tests.